Create Read Update Delete (CRUD) – Magento 2
- Bilal Malik
- Aug 7, 2017
- 2 min read
Updated: Aug 21, 2020
This tutorial is the continuation of create table tutorial, after creating table, we will create the Model for CRUD (Create Read Update Delete) operation.
I will explain crud operation for the below table structure

For CRUD operation you need to create three things.
1. Model
In Model you need to initialize resource model
app/code/Bilal/Cruddemo/Model/Cruddemo.php
<?php
namespace Bilal\Cruddemo\Model;
use Magento\Framework\Model\AbstractModel;
class Cruddemo extends AbstractModel
{
/**
* Define resource model
*/
protected function _construct()
{
$this->_init('Bilal\Cruddemo\Model\ResourceModel\Cruddemo');
}
}
2. Resource Model
In Resource model, you need to initialize table name and primary key.
app/code/Bilal/Cruddemo/Model/ResourceModel/Cruddemo.php
<?php
namespace Bilal\Cruddemo\Model\ResourceModel;
class Cruddemo extends \Magento\Framework\Model\ResourceModel\Db\AbstractDb
{
/**
* Define main table
*/
protected function _construct()
{
$this->_init('crud_test_emp1', 'id'); //here "crud_test_emp1" is table name, "id" is the primary key
}
}
3. Collection
In collection, you need to define Model and Resource Model.
app/code/Bilal/Cruddemo/Model/ResourceModel/Cruddemo/Collection.php
<?php
namespace Bilal\Cruddemo\Model\ResourceModel\Cruddemo;
class Collection extends \Magento\Framework\Model\ResourceModel\Db\Collection\AbstractCollection
{
/**
* Define model & resource model
*/
protected function _construct()
{
$this->_init(
'Bilal\Cruddemo\Model\Cruddemo',
'Bilal\Cruddemo\Model\ResourceModel\Cruddemo'
);
}
}
That’s it, now you can able to fetch table value in any block using dependency injection.
Get and Set Data in Block
Please note, we pass Bilal/Cruddemo/Model/CruddemoFactory in constructor, but none of the file found that location.
In Magento 2, each CRUD model has a corresponding factory class. All factory class names are the name of the model class, appended with the word “Factory”. So, since our model class is named Bilal/Cruddemo/Model/Bilal/Cruddemo this means our factory class is named Bilal/Cruddemo/Model/Bilal/CruddemoFactory
app/code/Bilal/Cruddemo/Block/Index/Index.php
<?php
namespace Bilal\Cruddemo\Block\Index;
class Index extends \Magento\Framework\View\Element\Template
{
protected $_cruddemo;
public function __construct(
\Magento\Framework\View\Element\Template\Context $context,
\Bilal\Cruddemo\Model\CruddemoFactory $cruddemo
)
{
$this->_cruddemo = $cruddemo;
parent::__construct($context);
}
public function addRecord($name, $age)
{
$data=array('name' => $name, 'age' => $age);
$this->_cruddemo->create()->setData($data)->save();
}
public function getValue()
{
$data=$this->_cruddemo->create()->getCollection();
foreach ($data as $d )
{
echo $d->getName().' - '; //table field "name"
echo $d->getAge().'<br>'; //table field "age"
}
}
}
after that, you can call this block method in your template file
app/code/Bilal/Cruddemo/view/frontend/templates/index/index.phtml
<?php
// add data
$block->addRecord('bilal',25);
echo "data added <br>";
// fetch data
$block->getValue();
?>
If you load the URL, one record gets added into our custom table and the page looks like
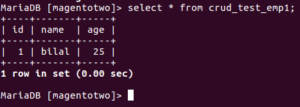

I hope it helps! Please carry the same source code to follow next tutorial.
コメント